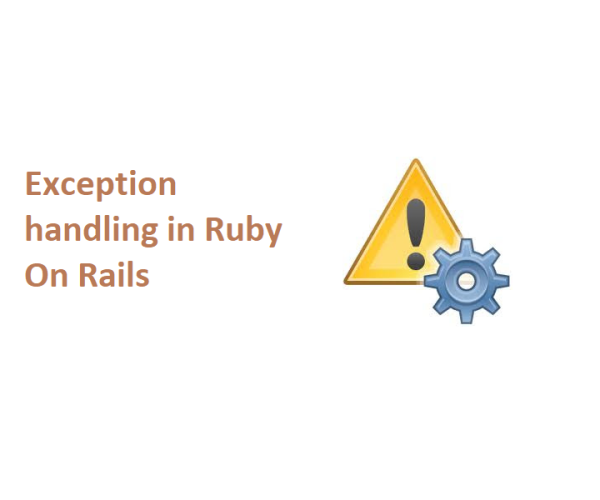
Mastering Custom Exception Handling in Ruby on Rails
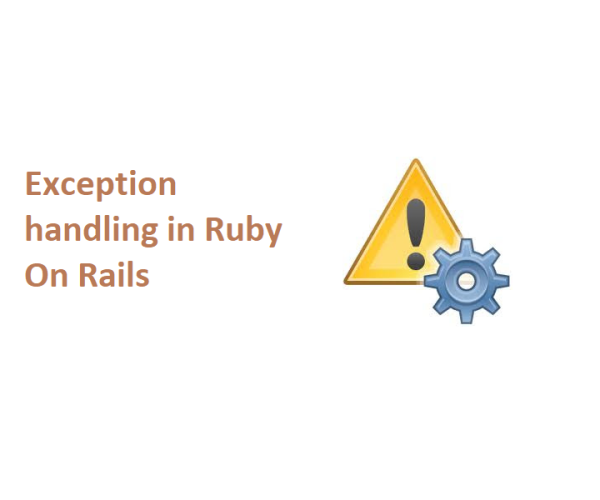
Custom exception handling in Ruby on Rails involves creating and using your own exception classes to handle specific error scenarios in a more controlled and organized manner. This approach allows you to define and raise exceptions that are tailored to the needs of your application.
Here's a detailed breakdown:
1. Define a Custom Exception Class:
Create a new class that inherits from StandardError or one of its subclasses. You can add an optional constructor to customize the error message.
class CustomError < StandardError
def initialize(message = 'Custom error message')
super(message)
end
end
2. Raise the Custom Exception:
In your code, raise the custom exception when a specific error condition occurs. This is typically done using the raise keyword.
raise CustomError.new('Something went wrong!')
3. Rescue the Custom Exception:
Use the begin, rescue, and end block to catch and handle the custom exception where needed. This provides a way to gracefully handle errors and take appropriate actions.
begin
# Code that might raise CustomError
raise CustomError.new('Something went wrong!')
rescue CustomError => e
# Handle the custom exception
puts "Custom Error: #{e.message}"
end
4. Use rescue_from in Controllers:
In Rails controllers, you can use the rescue_from method to handle specific exceptions globally for the entire controller or specific actions.
class ApplicationController < ActionController::Base
rescue_from CustomError, with: :handle_custom_error
def handle_custom_error(exception)
render plain: exception.message, status: :unprocessable_entity
end
end
This way, if a CustomError is raised anywhere in the controller, it will be caught by the handle_custom_error method.
5. Custom Logging:
Customize logging for your custom exceptions to aid in debugging. This ensures that relevant information about the exception is logged, helping you identify and address issues effectively.
rescue CustomError => e
Rails.logger.error "Custom Error: #{e.message}"
By implementing custom exception handling, you can create a hierarchy of exception classes, making your code more readable and maintainable. It also allows you to provide specific error responses for different scenarios, improving the overall robustness of your Ruby on Rails application.