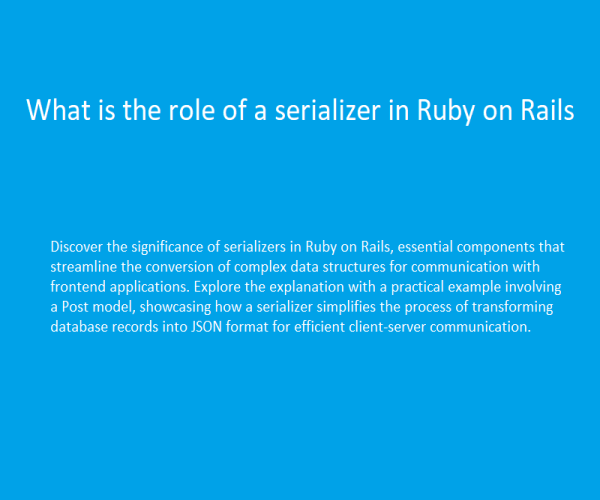
What is the role of a serializer in Ruby on Rails
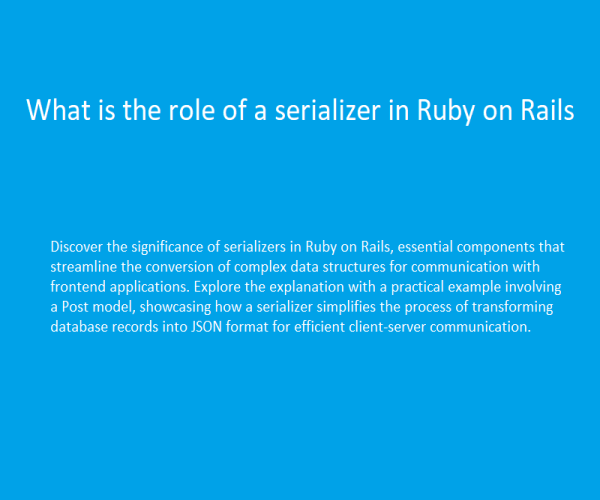
In Ruby on Rails (RoR), a serializer is a component that helps in converting complex data types, such as ActiveRecord models or Ruby objects, into a format that can be easily rendered into JSON or XML for communication between the server and the client. Serializers play a crucial role in turning complex data structures into a format that can be easily consumed by frontend applications.
Here's a simple explanation with an example:
Let's say you have a Rails model called Post with attributes like title and content. You want to send information about a post to a client application in JSON format. This is where a serializer comes into play.
Create a Serializer:
Start by creating a serializer for the Post model. Rails provides a built-in serializer through the Active Model Serializers gem.
# app/serializers/post_serializer.rb
class PostSerializer < ActiveModel::Serializer
attributes :id, :title, :content
end
In this example, the serializer defines that it should include the id, title, and content attributes of a Post object in the serialized output.
Use the Serializer:
In your controller or API endpoint, use the serializer to convert a Post instance into JSON.
# app/controllers/posts_controller.rb
class PostsController < ApplicationController
def show
@post = Post.find(params[:id])
render json: @post
end
end
By calling render json: @post, Rails will automatically use the PostSerializer to convert the @post object into JSON before sending the response.
JSON Output:
When a client makes a request to view a post, the response would look something like this:
{
"id": 1,
"title": "Example Post",
"content": "This is the content of the post."
}
The serializer has formatted the Post object into a JSON structure, making it easy for the client to parse and work with.