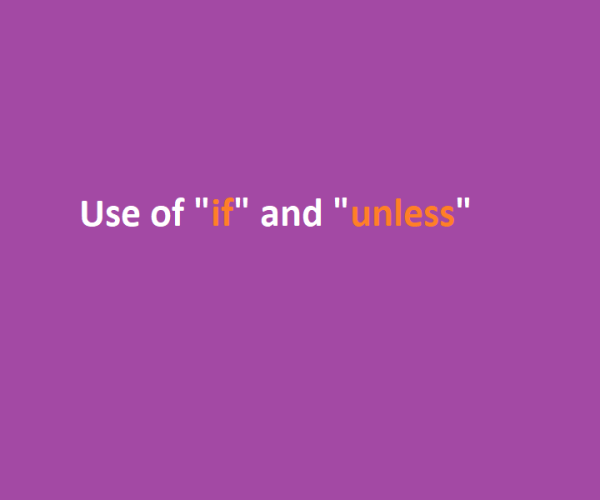
Exploring Conditional Logic in Ruby on Rails: Understanding the Use of 'if' and 'unless' Constructs
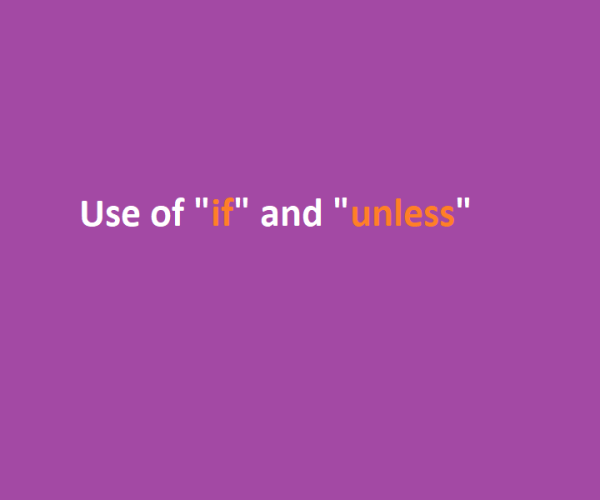
In Ruby on Rails (RoR), if and unless are conditional constructs used to control the flow of code execution based on certain conditions.
if: The if statement executes a block of code if a specified condition is true. It has the following syntax:
if condition
# code to execute if condition is true
end
Example:
if user_signed_in?
redirect_to dashboard_path
end
In this example, if the condition user_signed_in? evaluates to true, the code inside the if block (redirecting to the dashboard) will be executed.
unless: The unless statement is the opposite of if; it executes a block of code if a specified condition is false. It has the following syntax:
unless condition
# code to execute if condition is false
end
Example:
unless user_signed_in?
redirect_to login_path
end
In this example, if the condition user_signed_in? evaluates to false, the code inside the unless block (redirecting to the login page) will be executed.
Both if and unless can be used with modifiers to write more concise code. For example:
redirect_to dashboard_path if user_signed_in?
This line of code redirects to the dashboard path if the user is signed in. Similarly:
redirect_to login_path unless user_signed_in?
This line of code redirects to the login path unless the user is signed in.