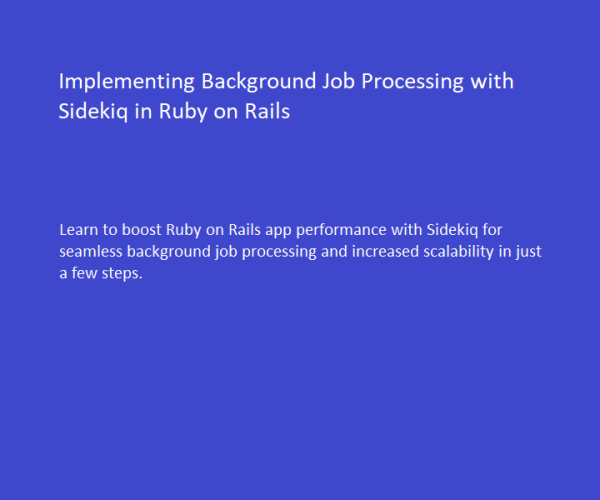
Implementing Background Job Processing with Sidekiq in Ruby on Rails
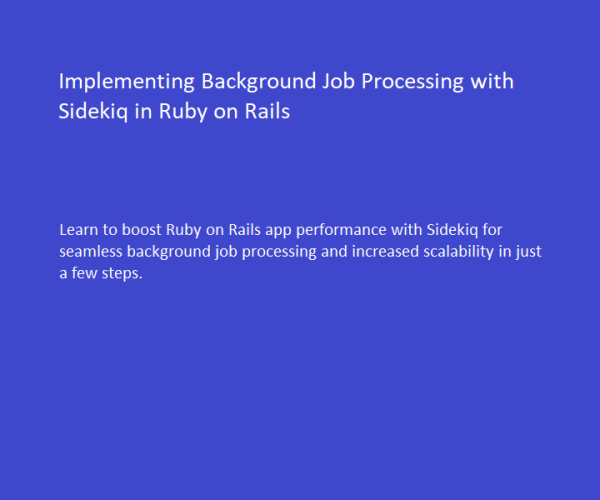
In Ruby on Rails (RoR), handling jobs asynchronously is often done using a background job processing system. One popular tool for this purpose is Sidekiq, which integrates seamlessly with Rails and uses Redis as a backend to manage the job queue. Below are the steps to set up a basic job handler using Sidekiq in a Rails application:
Add Sidekiq to your Gemfile:
Open your Gemfile and add the following line:
gem 'sidekiq'
Then run bundle install in your terminal to install the gem.
Configure Sidekiq:
Create a config/initializers/sidekiq.rb file and configure Sidekiq with Redis. For example:
# config/initializers/sidekiq.rb
Sidekiq.configure_server do |config|
config.redis = { url: 'redis://localhost:6379/0' }
end
Sidekiq.configure_client do |config|
config.redis = { url: 'redis://localhost:6379/0' }
end
Make sure to adjust the Redis URL based on your setup.
Create a background job:
Generate a new job using the following Rails command:
rails generate job ExampleJob
This will create a file in the app/jobs directory, typically named example_job.rb. Open this file and define the job:
# app/jobs/example_job.rb
class ExampleJob < ApplicationJob
queue_as :default
def perform(*args)
# Your background job logic goes here
puts "Example job performed with args: #{args.inspect}"
end
end
Enqueue the job:
Wherever you want to enqueue this job (e.g., in a controller or another job), you can do so like this:
ExampleJob.perform_later(arg1, arg2)
Replace arg1, arg2, etc., with the actual arguments you want to pass to the job.
Run Sidekiq:
Start the Sidekiq process in your terminal:
bundle exec sidekiq
This will start processing background jobs.
Now, whenever you call perform_later on an instance of ExampleJob, Sidekiq will enqueue the job and execute it asynchronously.